Pure mininamilist web app basics
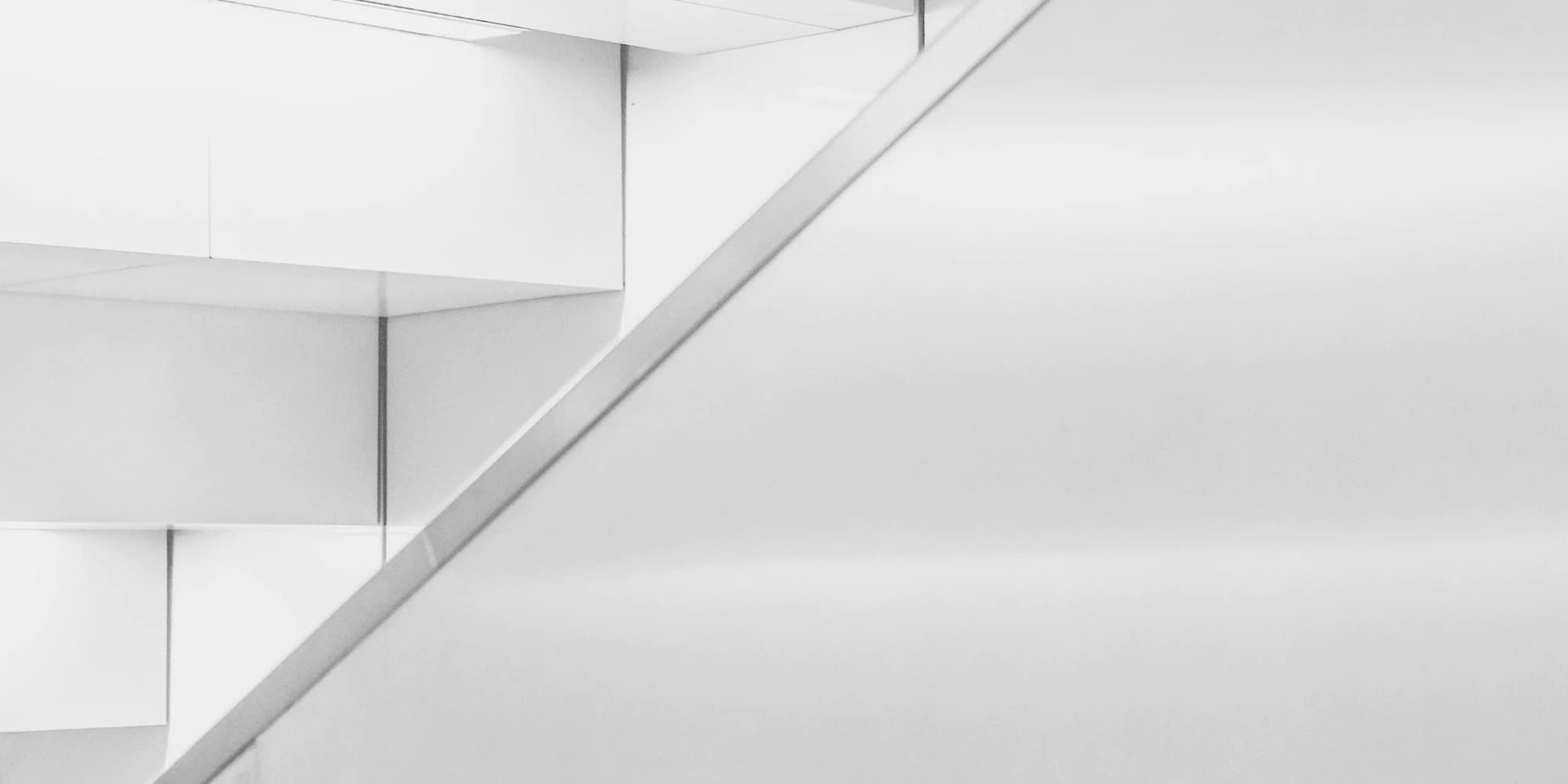
What are apps really made of?
You have a VScode project. It is a window into a directory on your computer.
How do we run code? - Runtime
How do we run code? We need a runtime to actually compile, compute, and execute the code.
Node
While developing locally, we'll probably be using Node as the runtime. Once we deploy our backend somewhere, we'll be using and/or configuring a runtime on the virtual machine that we deploy on.
If you're using node, you can test this out yourself -- you type 'node' (without the 's), and you'll see a message like: Welcome to Node.js v23.11.0. Type ".help" for more information. [>]
This is the Node REPL (Read Eval Print Loop). You are now 'inside' the Node runtime, in a state that lets you interact with the runtime. If we want to run a file, like a test file (test.js), we could go [>] require('./test.js)
Which would immediately run test.js for us.
The conventional and standard way to run code
It's inconvenient to enter the Node REPL and require your files manually. Sometimes the commands can get a bit long. We also might want to make it so our team uses the same commands to launch. We also should learn what the industry is doing as a whole.
Running NPM commands
So, we use Node Package Manager (npm) to handle the setting up of runtime and executing our code for us. In our terminal, we'll run
npm init
Which calls the 'npm' program (more specifically, the npm CLI), passing 'init' as a parameter, which will create a package.json file.
When we run other npm commands (i.e. npm install, npm run, Node will look for package.json, and handle your commands based on the contents of package.json).
Running scripts from package.json
The convention is to set up 'scripts' in our package.json. "scripts": { "test": "node test.js", "dev": "node src/index.js", "server": "node src/server.js" },
What this means is we'll type 'npm run' followed by whatever keys we put into the "scripts" object - i.e. we may type 'npm run test' into the command line.
Npm runs, looks for the relevant parameter in the scripts object, and then runs the actual command listed in the script.
Therefore, when we input into the CLI 'npm run test', the CLI will in turn run the command 'node test.js'.
Entry point into apps
Now that we understand how files get launched in the Node runtime, we can then understand that: The 'node' command is like an 'execute' command for our Javascript/Typescript scripts.
Therefore, if I have a file I want to run, I'll go into package.json and set 'node scriptToRun.js' in the "scripts" object, or I'll go 'node' -> 'require('./scriptToRun.js').
At this point, we can make simple programs such as
//.index.js
1 console.log('Hello')
We can then include "start": "node index.js"
Run in our terminal the command npm run start
And we'll see our index.js file execute!